Learning Intentions
Syllabus Outcome
SE-12-02 applies structural elements to develop programming code
Lesson Activity
BE2 – Working with Modules, File Systems, and HTTP
Introduction
In Node.js, modules are individual units of code that perform specific tasks and can communicate with other parts of your application. They are a key part of Node.js, allowing developers to split up complex programs into smaller, more manageable parts. This makes code more organised, reusable, and easier to maintain.
There are three main types of Node.js modules:
- Core Modules: These are built-in modules provided by Node.js, such as
http
,fs
, andpath
. You don’t need to install them as they come with Node.js. - Local (User-Defined) Modules: These are custom modules that you create yourself for specific purposes within your application.
- Third-Party Modules: These modules are developed by the community and can be installed via npm (Node Package Manager), allowing you to use additional functionality created by other developers.
By using these different types of modules, you can make your Node.js applications more efficient, well-structured, and capable of handling complex tasks with ease.
Source: Node.js Modules
Practical Tasks: Working with Node.js Modules, fs, and http
Make sure you’re working in the folder from the last lesson.
Goal:
In this lesson, you’ll:
- Create a custom module.
- Read from and write to a file using the
fs
(File System) module. - Build a basic web server using the
http
module.
By the end of this session, you’ll have a deeper understanding of how Node.js handles modules, files, and basic web servers.
Differences Between Python Imports and Node.js Exports
As you’re familiar with Python, you’re already comfortable with the concept of importing modules to keep your code organised and reusable. Node.js also lets you split your code into modules, but the way Node.js handles exports and imports has a few important differences from Python.
In Python, when you import a Python module, everything inside that module (functions, classes, variables) is automatically available to use unless you’ve hidden it. You just use the import
keyword or from ... import
for specific functions or classes.
For example, in Python:
# File: math_utils.py
def add(a, b):
return a + b
As you know, to use the add function in another file:
# File: main.py
from math_utils import add
print(add(2, 3)) # Output: 5
In Node.js, importing works a bit differently. To make functions or variables in one file available in another, you need to explicitly export them using exports
or module.exports
. Only what you export can be imported into other files.
For example, in Node.js:
// File: mathUtils.js
exports.add = function(a, b) {
return a + b;
};
To use this in another file, you would do the following:
// File: app.js
const mathUtils = require('./mathUtils');
console.log(mathUtils.add(2, 3)); // Output: 5
In Node.js, nothing from a file is automatically available to other files unless you explicitly export it.
Creating a Custom Module
Modules in Node.js help us break up our code into separate parts, making it easier to maintain and reuse.
- Open your existing project folder (from the previous lesson) in your terminal/command prompt.
- Create a new file named
greetings.js
using ‘code greetings.js’. This will act as our custom module. Inside this file, we’ll write two functions: one to say “Hello” and one to say “Goodbye.”
// greetings.js
exports.sayHello = function(name) {
return `Hello, ${name}!`;
};
exports.sayGoodbye = function(name) {
return `Goodbye, ${name}!`;
};
The keyword exports
is how we make functions or variables available outside of this file. Without exports
, the code inside this file would only be accessible here and couldn’t be used in other files.
- Create ‘
app.js
‘ using ‘code app.js‘. We’ll modify it to import and use this custom module above.
// app.js
const greetings = require('./greetings');
console.log(greetings.sayHello('Student'));
console.log(greetings.sayGoodbye('Student'));
The require()
function is used to load modules in Node.js. When we require('./greetings')
, we’re pulling in the functions we exported from greetings.js
and storing them in the greetings
variable. Now, we can use greetings.sayHello()
and greetings.sayGoodbye()
anywhere in our app.js
file.
- Run your app!
node app.js
By using modules, we’re separating our code into logical pieces. If your project grows, you can easily track and update individual modules without getting lost in a large file. It’s also helpful for reusing the same functionality in different parts of your project.
This is very similar to Python’s import functionality you would have used during your year 11 subjects.
Reading from and Writing to a File Using the fs Module
Node.js has a built-in File System (fs) module that allows you to interact with files on your system. You can read from, write to, or manipulate files in other ways.
Reading data from a file!
- In your project folder, create a new file called data.txt, then add the following to it:
This is a sample text file.
- Create a new JavaScript file called
fileOperations.js
. Here’s where we’ll write code to read fromdata.txt
and write new data to another file. - Add the following code to read the file:
const fs = require('fs');
// Reading the file
fs.readFile('data.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading the file:', err);
return;
}
console.log('File content:', data);
});
We’re using the fs.readFile()
method to read the contents of data.txt
. Notice that we passed 'utf8'
as the second argument to ensure that the file is read as plain text (instead of raw binary data). The method takes a callback function, which runs after the file is read. If there’s an error, it’s passed to err
, and if everything goes well, the file’s content is passed to data
.
- Run the script:
node fileOperations.js
Now, let’s write new data to a file!
- Update your fileOperations.js file to include code for writing data:
const fs = require('fs');
// Reading the file
fs.readFile('data.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading the file:', err);
return;
}
console.log('File content:', data);
// Writing new data to a file
const newData = `${data}\nAppended text!`;
fs.writeFile('newData.txt', newData, (err) => {
if (err) {
console.error('Error writing to the file:', err);
return;
}
console.log('Data successfully written to newData.txt');
});
});
After reading the content of data.txt, we’re appending new text and saving it to newData.txt. The fs.writeFile() function writes the content to the specified file, and if the file doesn’t exist, Node.js will create it for you.
- Run the file!
node fileOperations.js
This time, the program will read from data.txt
and write new content to newData.txt
.
Building a Basic Web Server Using the http Module
Node.js allows you to create web servers using the built-in http
module. This is powerful because you can handle web requests directly, without needing an external web server like Apache (what powers most web sites) or Nginx.
- In your project folder, create a file called server.js.
- Add the following code to server.js to create a basic web server:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.write('Hello, welcome to my Node.js server!');
res.end();
});
server.listen(3000, () => {
console.log('Server is listening on port 3000');
});
We use the http.createServer() method to create a simple web server. The server listens for requests (such as when you visit a URL in a browser) and responds with a plain text message. The res.writeHead() method sets the HTTP response status and headers, and res.write() sends the body of the response.
- Run your server
node server.js
- You should see:
Server is listening on port 3000
- Open your browser and go to http://localhost:3000. You should see:
Hello, welcome to my Node.js server!
By using the http module, you can create web servers directly in Node.js, giving you full control over how your application handles incoming requests and responds to users.
Bonus Activity
If you have time to spare, curious, or just want to learn more, you can follow the steps in the video below to create your very own Node.js weather application.
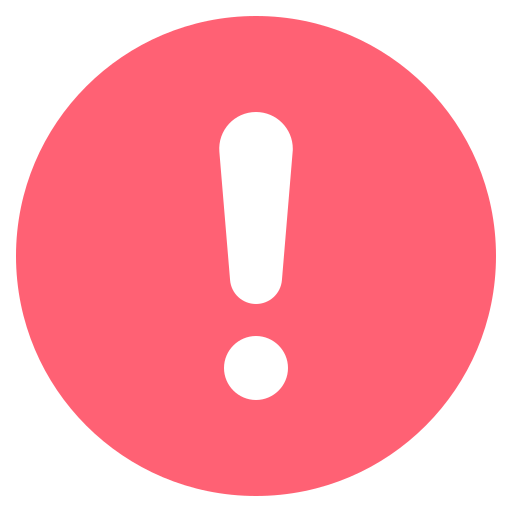
Next lesson in part 3, we go through steps on how we use and integrate SQLite with Node.js