Learning Intentions
- Understand how to create and interact with relational databases using SQL for basic CRUD operations.
- Learn how Object-Relational Mapping (ORM) simplifies database management by abstracting SQL queries into programming language commands.
- Compare the direct use of SQL with ORM techniques and understand the advantages and limitations of each approach.
Syllabus Outcome
SE-12-06: Justifies the selection and use of tools and resources to design, develop, manage, and evaluate software.
Lesson Activity
HSC Style Questions
Introduction to SQL
You will begin the lesson by watching the video “MySQL – The Basics // Learn SQL in 23 Easy Steps – YouTube“. This video introduces foundational SQL concepts that will need to understand before diving into Object-Relational Mapping (ORM).
During this video, you will:
- Learn how to create and interact with relational databases using SQL.
- Understand how to structure databases with tables, datatypes, and constraints.
- Perform basic CRUD (Create, Read, Update, Delete) operations using SQL queries.
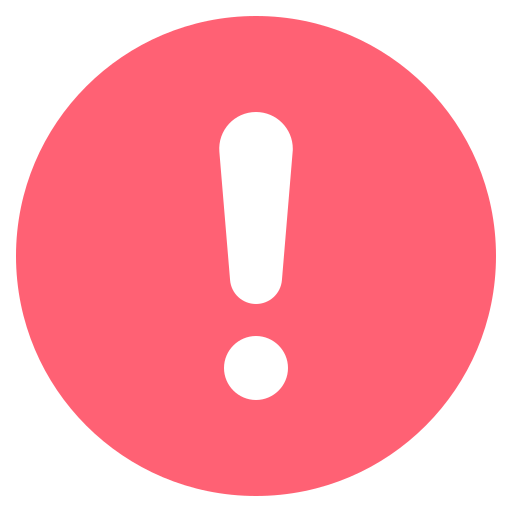
Part of the video will require the installation of MySQL Community Server and MySQL Workbench. I suggest downloading those while watching the video early on.
Do not use the suggested “MySQL Installer for Windows“, as it as not been updated to support the newer version of MySQL.
As you watch the video, pay attention to the demonstration of how SQL commands are written and executed in a database environment. These concepts are foundational, and they will help you compare the direct use of SQL with ORM techniques in the next part of the lesson.
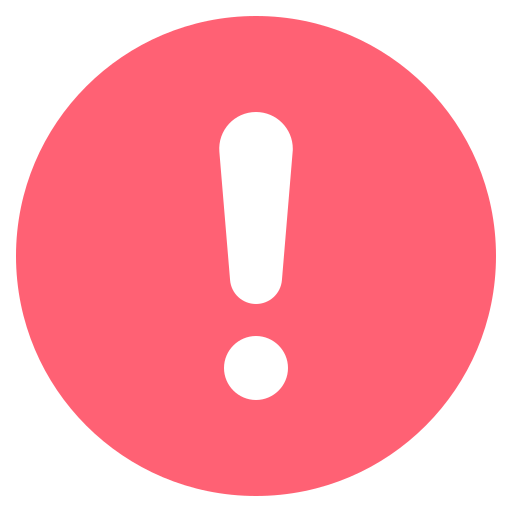
Learn SQL: Queries Cheatsheet | Codecademy
Codecademy provides has excellent cheatsheet you can print out and use when working with SQL. You can also download it here:
Transition into Object-Relational Mapping (ORM)
Now that you’ve experienced how SQL interacts directly with a database through the video tutorial, let’s explore a different approach to managing databases that integrates seamlessly with modern programming languages: Object-Relational Mapping (ORM).
ORMs are libraries or frameworks that allow you to work with databases in an object-oriented way, meaning you don’t have to write SQL manually every time. Instead, you use the programming language you’re already working in, like Python or JavaScript, to interact with the database. The ORM translates your high-level programming language commands into SQL under the hood.
Let’s break down how ORM compares to writing SQL directly and why ORM is such a popular approach in web development today.
SQL vs ORM
When you write SQL directly, you’re speaking to the database in its native language. You tell the database exactly what to do using commands like SELECT
, INSERT
, UPDATE
, and DELETE
. For example, if you want to add a new user to the database, you might write:
INSERT INTO users (name, age) VALUES ('Ben', 40);
With an ORM, however, you stay within the comfort of your programming language. Instead of writing SQL, you might do something like this in Python with an ORM like SQLAlchemy:
new_user = User(name='Ben', age=40)
session.add(new_user)
session.commit()
Alternatively, with an ORM like Sequelize in Node.js, it would look like this:
const ben = await User.create({
name: 'Ben',
age: 40,
});
const users = await User.findAll();
It looks like regular object-oriented code, right? That’s because ORMs are designed to bridge the gap between your code and your database. You don’t have to worry about SQL syntax or writing repetitive queries. ORMs handle that for you.
Why Choose ORM?
Now, you might be wondering why you should use an ORM when you already know SQL. After all, SQL is powerful and gives you full control over your database interactions. Well, here’s where ORMs shine:
- Simplified Code: ORMs allow you to write less boilerplate code. Instead of repeating SQL queries for every CRUD operation, you work with objects and methods in your programming language.
- Database Abstraction: ORMs provide a layer of abstraction between your application and the database. This means if you switch from, say, MySQL to PostgreSQL or even SQLite, you won’t need to rewrite your queries. The ORM handles these differences for you.
- Security: ORMs often come with built-in protections against SQL injection attacks. This means your application is less vulnerable to malicious users who might try to manipulate your SQL queries.
Let’s pause here and think about a scenario: You’re building an e-commerce platform. You have a table for products, users, and orders. Writing SQL queries for every product and user interaction could quickly lead to hundreds of lines of SQL code. By using an ORM, however, you could dramatically reduce this complexity by working with objects like Product
, User
, and Order
, making your code more maintainable and readable.
Source: What is an ORM – The Meaning of Object Relational Mapping Database Tools | What is object-relational mapping (ORM)? – TechTarget Definition
Performance Considerations: Is ORM Slower?
You might have heard that ORMs are slower than SQL, and in some cases, that’s true. The extra layer of abstraction that makes ORMs easy to use can add some overhead. However, in most web applications, this performance difference is negligible and far outweighed by the benefits of cleaner, more maintainable code. That said, when performance is critical, you can always write raw SQL queries alongside your ORM code for those specific cases.
Source: Why ORMs are slow (and getting slower) | EdgeDB Blog
Popular ORMs in Different Languages
To give you a sense of what’s available, here are some of the most widely used ORM frameworks:
- Python: SQLAlchemy and Django ORM
- JavaScript/Node.js: Sequelize
- Ruby: ActiveRecord (used in Ruby on Rails)
- Java: Hibernate
Each of these frameworks allows developers to interact with relational databases through their language’s object-oriented features, making database operations feel like an integrated part of the language itself.
Source: What is an ORM – The Meaning of Object Relational Mapping Database Tools
To end this lesson, you are going to answer some HSC style questions and submit them to me for marking. Consider this an open-book exam, and as you might not be completely familiar with SQL right away, you will find help with the last question by reviewing this section in the video from the start of the lesson.