Learning Intentions
- Understand JavaScript’s basics and its role in web development
- Explore JavaScript’s importance in creating Progressive Web Apps (PWAs)
- Gain practical JavaScript skills through examples and resources
Syllabus Outcome
SE-12-03: analyses how current hardware, software and emerging technologies influence the development of software engineering solutions
Lesson Activity
JavaScript W3C Research / Activities
Introduction
JavaScript is a lightweight, cross-platform programming language primarily used to create interactive and dynamic elements on web pages. Introduced by Netscape in 1995, it has since become one of the core technologies of the web, alongside HTML and CSS. JavaScript is known for its versatility, enabling both client-side and server-side development. On the client side, JavaScript interacts with the browser’s Document Object Model (DOM) to create interactive features, such as form validation, animations, and real-time content updates.
Features
JavaScript has several key features that make it an indispensable tool for web developers:
- Event-driven programming: JavaScript allows developers to respond to user actions, such as clicks, form submissions, or keyboard inputs, enhancing user experience.
- Dynamic typing: It is a weakly typed language, meaning that variables can hold different data types without the need for explicit declarations.
- Cross-platform compatibility: JavaScript runs in all modern web browsers, making it highly accessible for developing interactive web pages.
- Extensibility: With frameworks and libraries like React, Angular, and Vue.js, developers can create powerful and scalable web applications.
Why Learn JavaScript?
Learning JavaScript is essential for any aspiring web developer because it is the language that brings websites to life. Without JavaScript, web pages would be static and lack interactivity. From adding simple effects like hover states to developing complex web applications, JavaScript is the foundation for modern, dynamic websites. Its growing ecosystem, including popular frameworks and extensive community support, ensures that JavaScript skills are highly sought after in the industry.
Sources:
Comparing JavaScript to Python
You will already be familiar with Python, so here are some key similarities and differences:
Similarities:
- Both are high-level, interpreted languages
- Both support functional and object-oriented programming styles
- Many control structures are similar (if statements, for loops, while loops)
Differences:
- JavaScript uses curly braces
{}
to define code blocks, while Python uses indentation - JavaScript uses
var
,let
, orconst
to declare variables, while Python doesn’t require declaration - JavaScript uses
function
keyword or arrow notation=>
to define functions, while Python usesdef
Python vs JavaScript Example
def greet(name):
print("Hello, " + name + "!")
greet("Alice")
function greet(name) {
console.log("Hello, " + name + "!");
}
greet("Alice");
JavaScript Examples
W3Schools have a collection of examples where you can see the impact JavaScript can have on various elements of a website.
JavaScript in Progressive Web Apps
We learnt about PWA’s back in the very first lesson, but as a refresher, Progressive Web Apps (PWAs) are web applications that use modern web capabilities to deliver an app-like experience to users. They combine the best of web and native applications, offering features such as offline functionality, push notifications, and home screen installation, while maintaining the reach and accessibility of web applications.
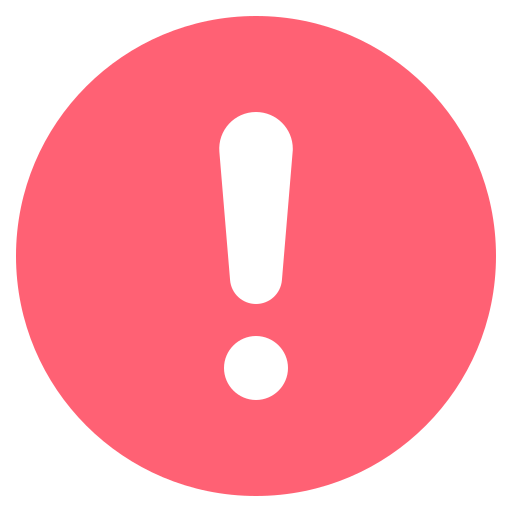
Once you have started learning JavaScript, all of the information below will make more sense.
JavaScript’s Role in PWAs
Below are some examples of JavaScript interacting with PWA elements.
Service Workers
- JavaScript files that run separately from the main browser thread
- Enable offline functionality and background syncing
- Example: Caching assets for offline use
self.addEventListener('install', function(event) {
event.waitUntil(
caches.open('my-cache').then(function(cache) {
return cache.addAll([
'/',
'/styles/main.css',
'/script/main.js'
]);
})
);
});
Source: Get started with Progressive Web Apps – Microsoft Edge Developer documentation | Microsoft Learn
Push Notifications
After enabling push notifications through Service Workers and the Push API, you can send a notification.
Notification.requestPermission().then(function(result) {
if (result === 'granted') {
navigator.serviceWorker.ready.then(function(registration) {
registration.showNotification('Notification Title', {
body: 'This is the notification body',
icon: '/images/icon.png',
vibrate: [200, 100, 200]
});
});
}
});
Source: How to make PWAs re-engageable using Notifications and Push – Progressive web apps | MDN
Lesson Activity
JavaScript is a very versatile language that is used in a wide range of applications/areas, including Web Development (Front-End), Server-Side Development, Mobile App Development, Desktop Application Development, Game Development, Web Servers and APIs, Web Assembly, Browser Extensions, Automation and Scripting, Internet of Things (IoT).
That makes the path to learning JavaScript a very specific one, so the learning that needs to be done will be completely up to you.
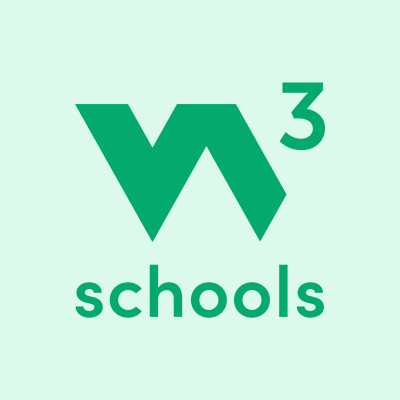
First, start by looking at W3Schools, ‘JavaScript Where To‘. This page explains the different ways to get started with implementing JavaScript into a website.
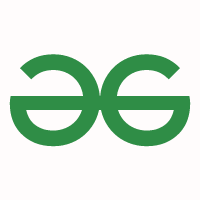
Second, as a experienced Python programmer who has a solid grasp of programming fundimentals, you can make yourself familiar more with JavaScript by looking over GeeksforGeeks guide, ‘JavaScript Cheat Sheet – A Basic Guide to JavaScript‘.
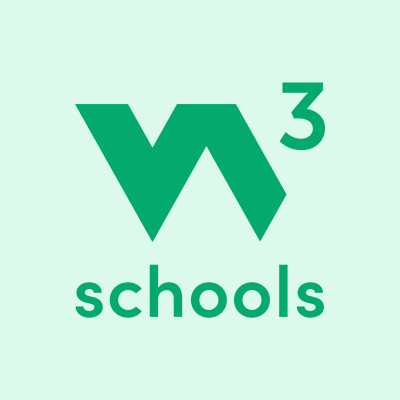
Third, learn about how JavaScript can interact with the browsers Document Object Model of the page. W3Schools guide, ‘JavaScript HTML DOM‘, will teach you how to change the content of HTML elements, CSS elements, react to HTML DOM events, and add/delete HTML elements.